UI Components
TextField
UI component for single-line text entry.
<TextField>
is a UI component for single-line text entry.
For multi-line text input, see TextView.
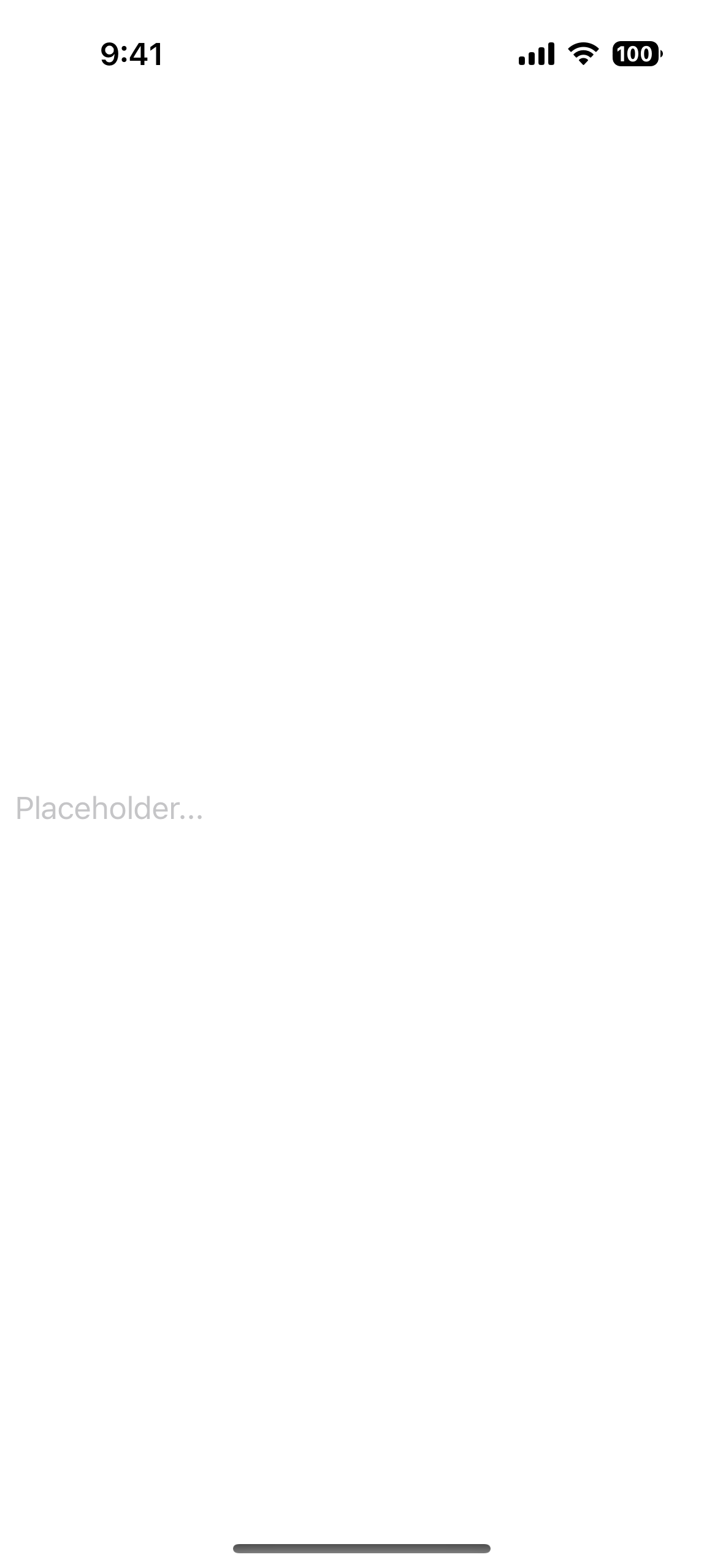
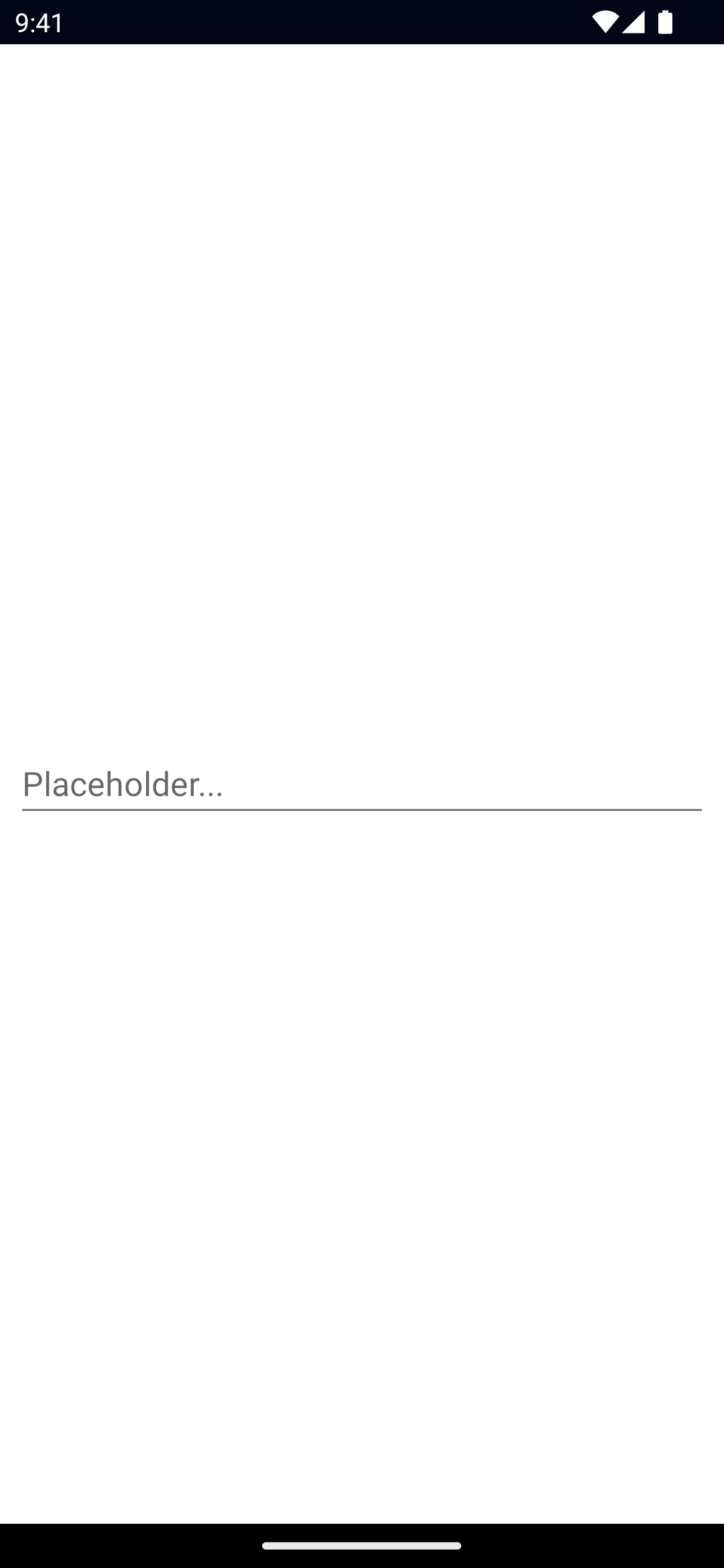
<TextField hint="Placeholder..." />
Examples
Formatting text inside a TextField
If you need to style parts of the text, you can use a combination of a FormattedString
and Span
elements.
<TextField>
<FormattedString>
<Span text="This text has a " />
<Span text="red " style="color: red" />
<Span text="piece of text. " />
<Span text="Also, this bit is italic, " fontStyle="italic" />
<Span text="and this bit is bold." fontWeight="bold" />
</FormattedString>
</TextField>
Props
text
text: string
Gets or sets the text
of the TextField.
hint
hint: string
Gets or sets the placeholder text for the TextField.
editable
editable: boolean
When set to false
the TextField is read-only.
Defaults to true
.
keyboardType
keyboardType: CoreTypes.KeyboardType | number // "datetime" | "email" | "integer" | "number" | "phone" | "url"
Gets or sets the keyboard type shown when editing this TextField.
On iOS, any valid UIKeyboardType
number works, for example:
keyboardType = 8 // UIKeyboardType.DecimalPad
See CoreTypes.KeyboardType, UIKeyboardType.
returnKeyType
returnKeyType: CoreTypes.ReturnKeyType // "done" | "go" | "next" | "search" | "send"
Gets or sets the label of the return key.
isEnabled
Allows disabling the TextField. A disabled TextField does not react to user gestures or input.
Default value is true
.
maxLength
maxLength: number
Limits input to the specified number of characters.
secure
secure: boolean
Hides the entered text when true
. Useful for password input fields.
Defaults to false
.
secureWithoutAutofill
secureWithoutAutofill: boolean
Prevents iOS 12+ auto suggested strong password handling. iOS only
Defaults to false
.
autocapitalizationType
autocapitalizationType: CoreTypes.AutocapitalizationType // "allCharacters" | "none" | "sentences" | "words"
Gets or sets the autocapitalization type.
See CoreTypes.AutocapitalizationType
...Inherited
For additional inherited properties, refer to the API Reference.
Methods
focus()
focus(): boolean
Focuses the TextField and returns true
if the focus was succeessful.
dismissSoftInput()
dismissSoftInput(): void
Hides the on-screen keyboard.
Events
textChange
on('textChange', (args: PropertyChangeData) => {
const textField = args.object as TextField
console.log('TextField text changed:', args.value)
})
Emitted when the input text changes.
Event data type: PropertyChangeData
returnPress
on('returnPress', (args: EventData) => {
const textField = args.object as TextField
console.log('TextField return key pressed.')
})
Emitted when the return key is pressed.
focus
on('focus', (args: EventData) => {
const textField = args.object as TextField
console.log('TextField has been focused')
})
Emitted when the TextField gains focus.
blur
on('blur', (args: EventData) => {
const textField = args.object as TextField
console.log('TextField has been blured')
})
Emitted when the TextField loses focus.
Native component
- Android:
android.widget.EditText
- iOS:
UITextField